About NetSquid
The Network Simulator for Quantum Information using Discrete events (NetSquid) is a software tool for the modelling and simulation of scalable quantum networks developed at QuTech. The goal of NetSquid is to enable scientists and engineers to design the future quantum internet as well as modular quantum computing architectures.
One of NetSquid’s key features is its ability to easily and accurately model the effects of time on the performance of quantum network and quantum computing systems. This forms an essential ingredient in developing scalable systems which require a design that can mitigate the limited lifetime of quantum bits processed by quantum devices.
Read More
NetSquid’s modular approach allows a detailed physical modeling of individual components like lego blocks that can easily be assembled to form complex simulations of large scale systems by connecting together different lego blocks. Example use cases of NetSquid include:
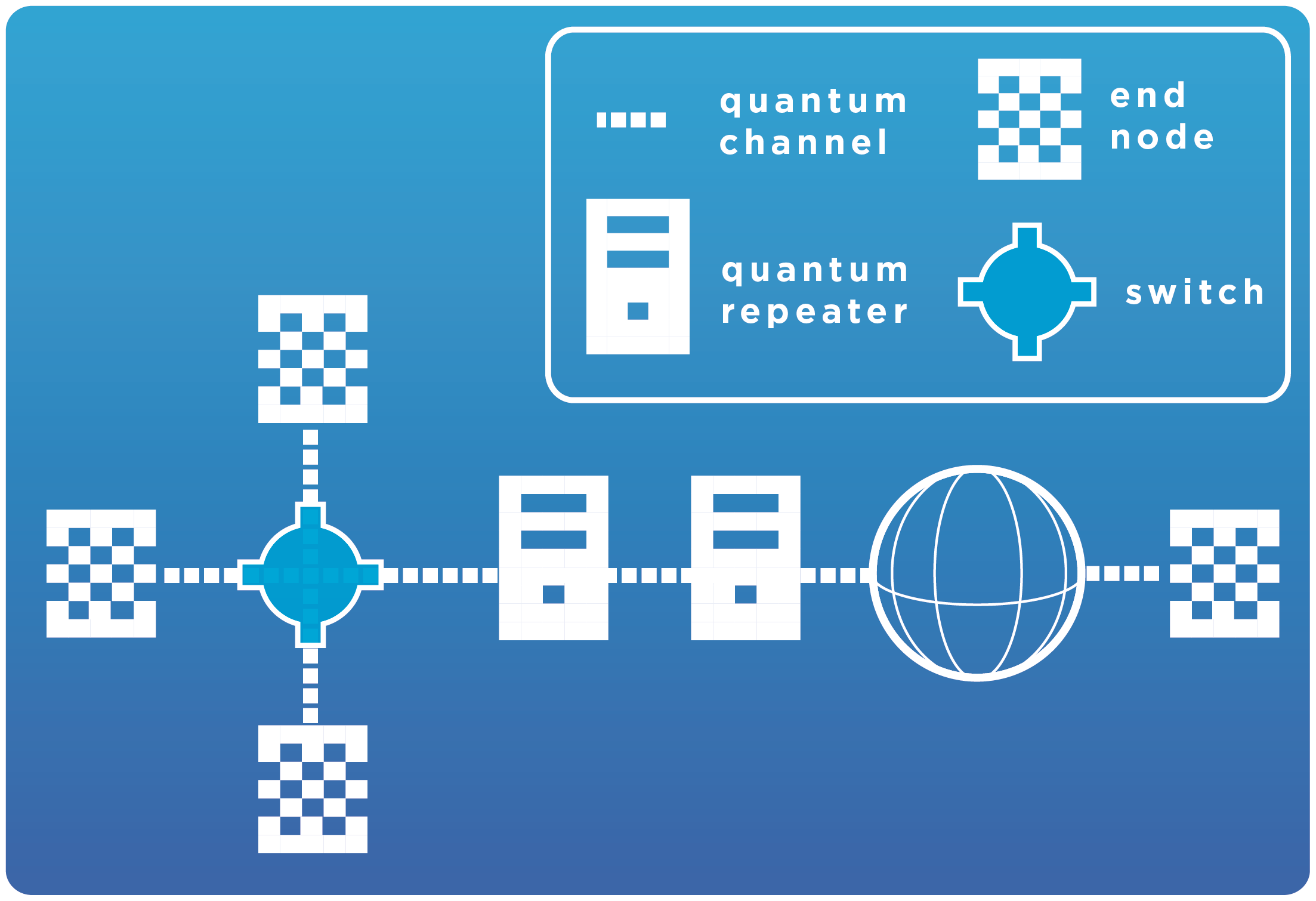
More detailed information about NetSquid is available in our paper.
Please cite this paper if you use NetSquid in your research.
Read MoreBibliography entry for the NetSquid paper (BibTex format):
@article{netsquid,
title={NetSquid, a discrete-event simulation platform for quantum networks},
author={Coopmans, Tim and Knegjens, Robert and Dahlberg, Axel and Maier, David and Nijsten, Loek and de Oliveira Filho, Julio and Papendrecht, Martijn and Rabbie, Julian and Rozpedek, Filip and Skrzypczyk, Matthew and Wubben, Leon and de Jong, Walter and Podareanu, Damian and Torres-Knoop, Ariana and Elkouss, David and Wehner, Stephanie},
journal={Commun Phys 4, 164},
year={2021},
doi={10.1038/s42005-021-00647-8},
archivePrefix={arXiv},
eprint={2010.12535},
primaryClass={quant-ph}}
Key Features of NetSquid
Discrete Event Simulation
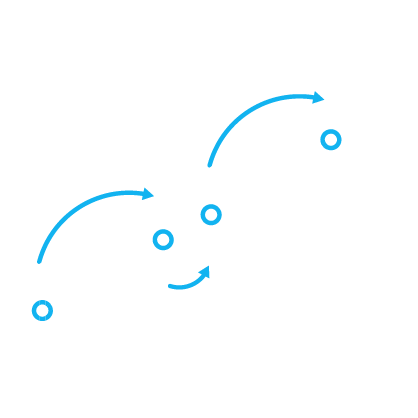
Discrete Event Simulation
Quantum Computation Library
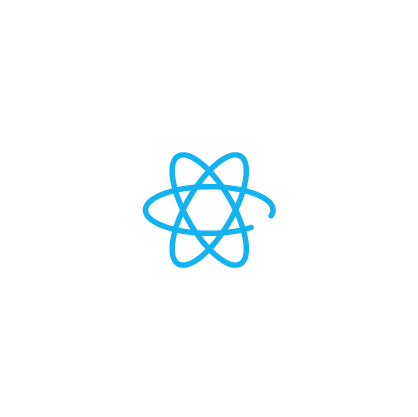
Quantum Computation Library
Multiple Quantum State Formalisms
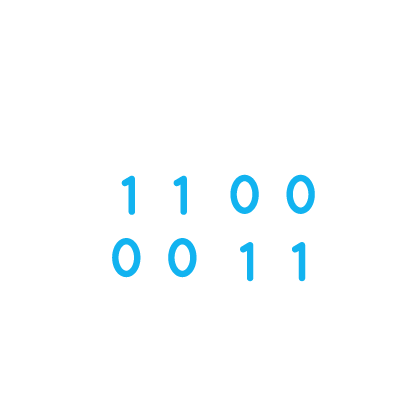
Multiple Quantum State Formalisms
Physically Realistic Building Blocks
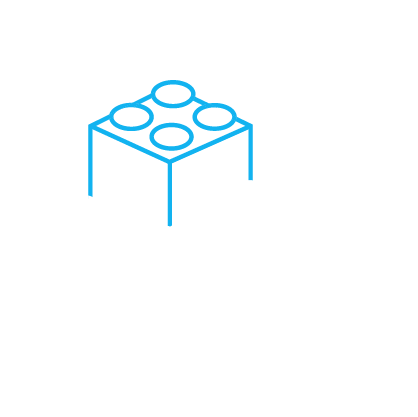
Physically Realistic Building Blocks
Available as Python Package
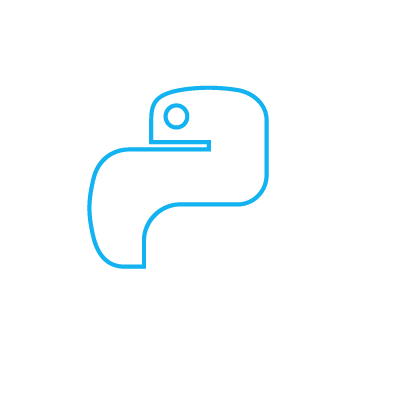
Available as Python Package
Asynchronous Framework
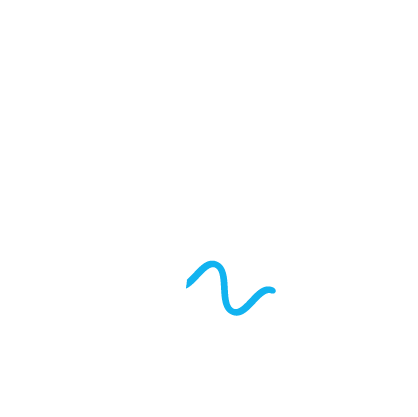
Asynchronous Framework
Getting Started with NetSquid
- NetSquid is free to download and use! To get access please agree to the terms of service by registering via the community forum:
-
NetSquid is a package for the Python 3 programming language. It can be installed directly from our package server using the Python package installer and your forum credentials:
pip3 install --extra-index-url https://pypi.netsquid.org netsquid
-
To get started visit the online documentation (requires registration) to learn the basics using the NetSquid in 10 minutes guide or to dive into the complete tutorial.
- The community forum is a great place to find answers to NetSquid related questions and interact with the development team and other users.
Code example: a game of quantum ping-pong
- >>> import netsquid as ns
- >>> from netsquid.components import QuantumChannel
- ...
- >>> class PingPongProtocol(ns.protocols.NodeProtocol):
- ... def run(self):
- ... port = self.node.ports['qubitIO']
- ... while True:
- ... yield self.await_port_input(port)
- ... qubit = port.rx_input().items[0]
- ... observable = ns.X if self.node.name == 'Bob' else ns.Z
- ... outcome, _ = ns.qubits.measure(qubit, observable)
- ... print(f'{ns.sim_time()}: {self.node.name} measured '
- ... f'{outcome} in {observable.name} basis')
- ... port.tx_output(qubit)
- ...
- >>> network = ns.nodes.Network(
- ... 'PingPongNetwork', nodes=['Alice', 'Bob'])
- >>> network.add_connection('Alice', 'Bob',
- ... channel_to=QuantumChannel('A2B', delay=10),
- ... channel_from=QuantumChannel('B2A', delay=10),
- ... port_name_node1='qubitIO', port_name_node2='qubitIO')
- >>> proto_a = PingPongProtocol(network.nodes['Alice']).start()
- >>> proto_b = PingPongProtocol(network.nodes['Bob']).start()
- >>> qubit = ns.qubits.qubitapi.create_qubits(1)
- >>> network.nodes['Alice'].ports['qubitIO'].tx_output(qubit)
- >>> ns.sim_run(duration=50)
- 10.0: Bob measured 1 in X basis
- 20.0: Alice measured 1 in Z basis
- 30.0: Bob measured 0 in X basis
- 40.0: Alice measured 1 in Z basis
NetSquid In Action
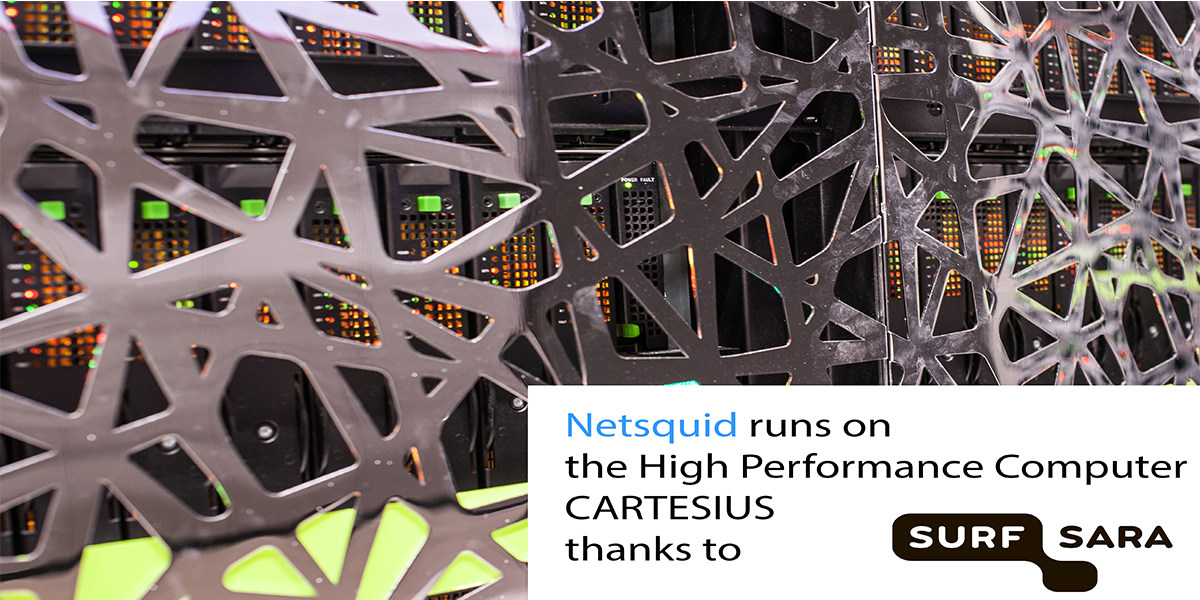
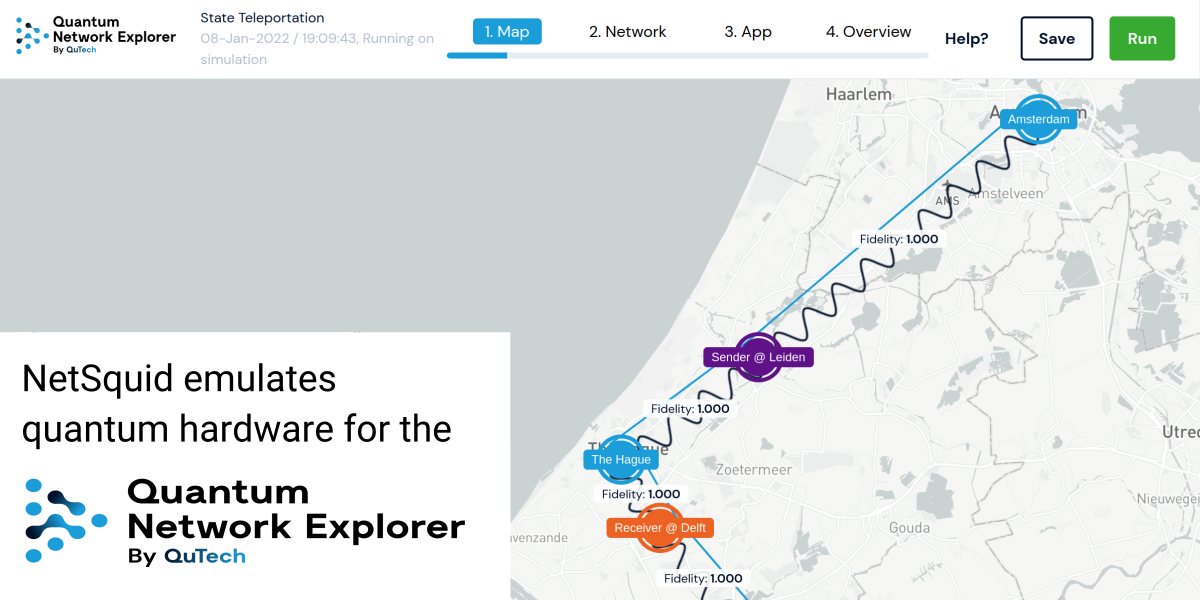
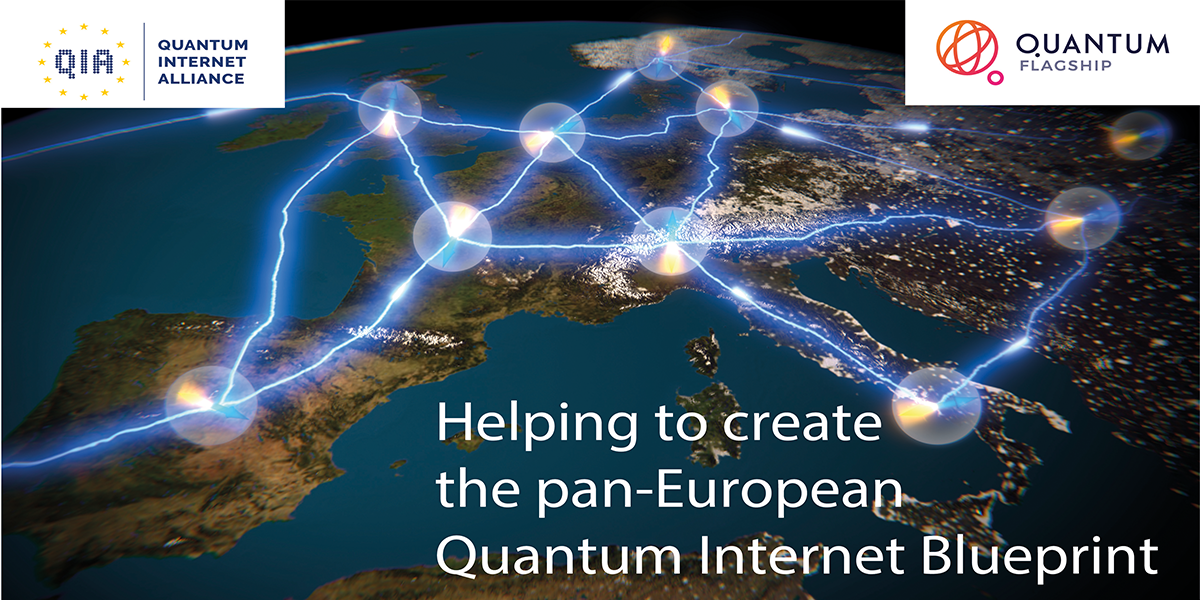
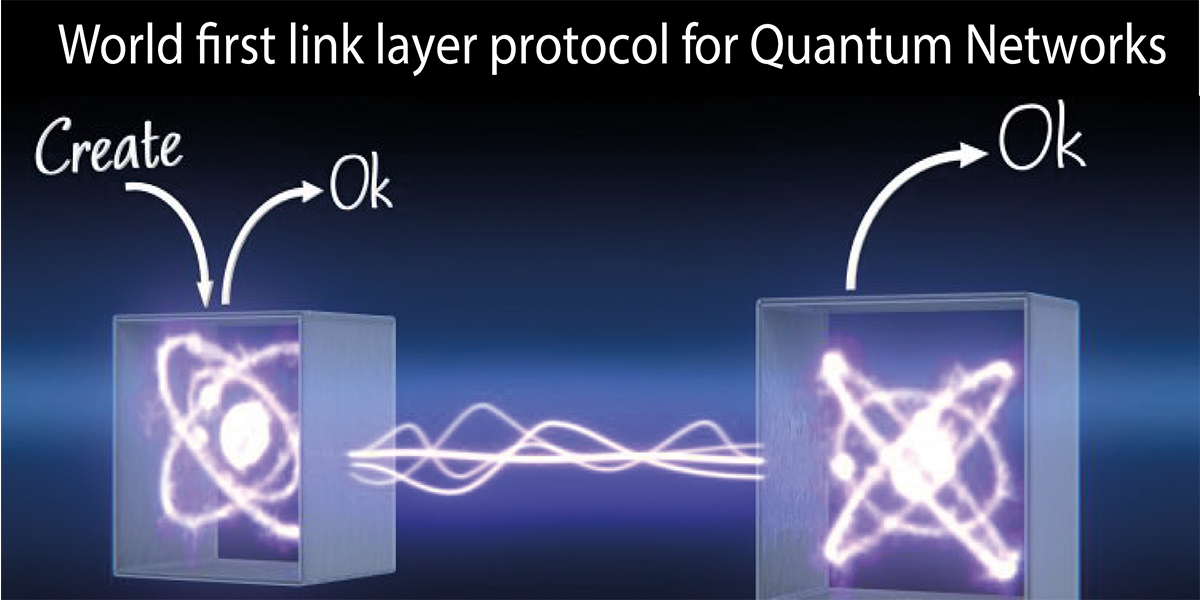